419 Page Expired Error in Laravel - How to fix it?
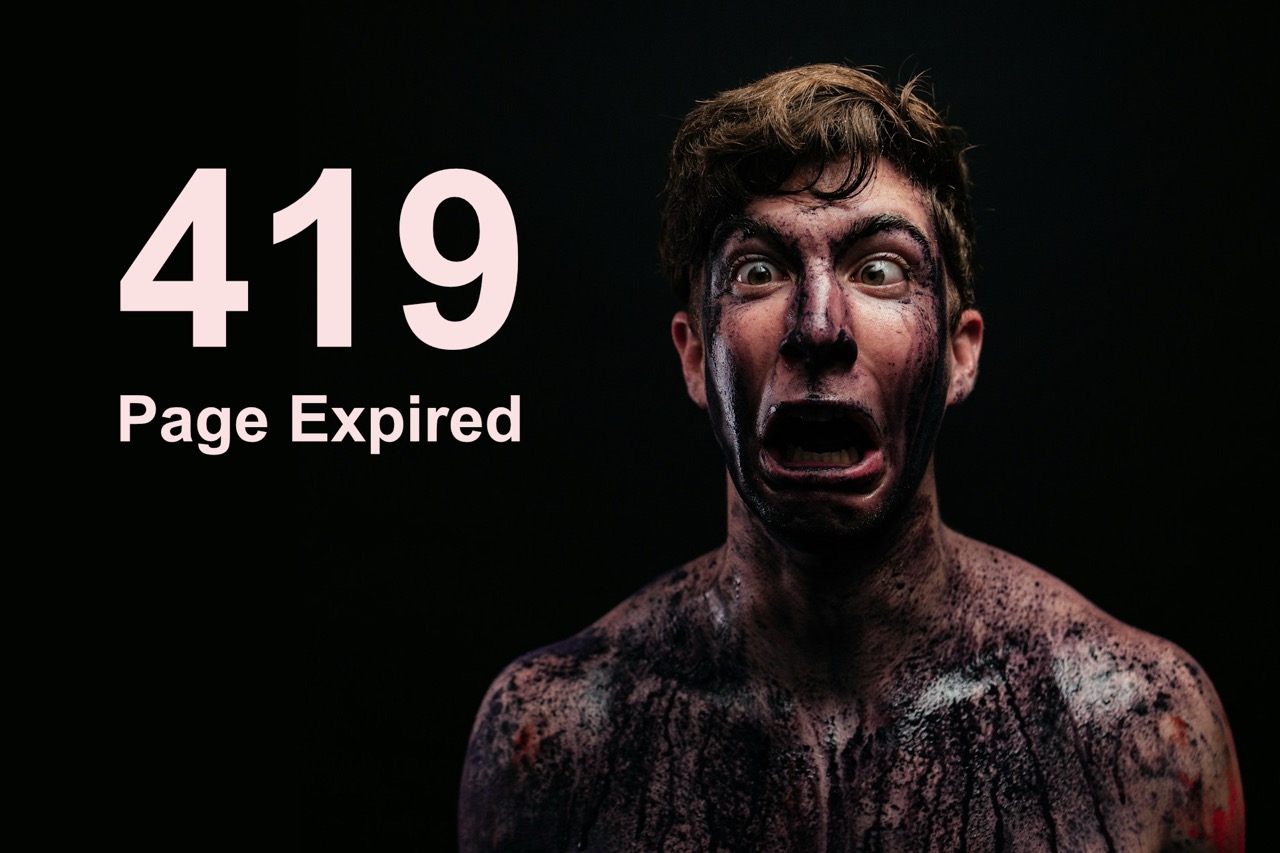
In the world of Laravel applications, encountering the “Page Expired” error with HTTP code 419 can be a common source of frustration. This error is generally linked to issues with Cross-Site Request Forgery (CSRF) tokens, an essential security feature in Laravel.
This article dives into why the “419 Page Expired” error occurs, how to address it, and some best practices to help keep it from happening in the first place.
Why Does the “419 Page Expired” Error Occur?
The 419 Page Expired error is typically a CSRF token mismatch issue. CSRF tokens protect your application from unauthorized form submissions by verifying that requests are being made by authenticated users within the application.
In Laravel, you often use the @csrf directive in forms, which generates a unique, hidden CSRF token for each form session.
Common causes for this error:
-
Token Expiration: CSRF tokens have a limited lifespan for security reasons. Leaving a page with a form open too long (e.g., login pages) can cause the token to expire, resulting in a 419 error when the form is eventually submitted.
-
Missing CSRF Directive in Forms: If you forget to include the @csrf directive in your form, Laravel will block the request because it expects a valid CSRF token, which the VerifyCsrfToken middleware checks for every POST, PUT, PATCH, and DELETE request.
-
Session Expiration: The CSRF token is tied to the user’s session. If the session expires, the token is invalidated, causing the form submission to fail.
-
Cookie or Session Configuration Issues: Misconfigurations in cookie or session settings, such as improper domain configuration, can disrupt session handling and prevent Laravel from correctly matching CSRF tokens.
- Mixing Https with Http: When your application serves request via HTTPS and you try to access it via HTTP, you might encounter this error.
Other Potential Causes:
- Browser Cache or Cookies: Sometimes, outdated cookies or cached data may interfere with CSRF validation.
- Server Configuration: If your server setup doesn’t handle sessions properly (for instance, if it loses session data), this can lead to 419 errors as well.
How to Resolve the “419 Page Expired” Error
To tackle this error effectively, follow these troubleshooting steps:
- Always Include the CSRF Token
Use the @csrf directive in all your forms to ensure CSRF protection is properly in place:
<form action="/submit" method="POST"> @csrf <!-- form fields here --> </form>
- Check Session and Cookie Configuration
Verify your session and cookie settings in the config/session.php file
- Set a secure and appropriate session driver, such as file, cookie, or database.
- Ensure the SESSION_DOMAIN is set correctly if your application uses subdomains, which can cause session misconfigurations.
- Make sure you're using the correct protocol (HTTPS vs HTTP) when opening your application.
- Refresh Expired Sessions
If users frequently encounter 419 errors due to sessions expiring, consider extending the session lifetime (in session.lifetime in config/session.php). Be cautious, however, as extending the session lifetime too much can expose the application to security risks. - Clear Browser Cache and Cookies
In cases where clearing configurations doesn’t resolve the issue, try instructing users to clear their browser cache and cookies, as these can sometimes hold onto outdated CSRF tokens. - Server-Side Debugging
If you still experience issues, check your server configuration to ensure sessions are handled consistently and reliably. Also, make sure the storage paths (e.g., for session files) have proper permissions and are not clearing unexpectedly.
Disabling CSRF Protection on Specific Routes
In some cases, you may want to exclude specific routes from CSRF protection, especially when integrating with external services that don’t use CSRF tokens. Instead of removing the CSRF middleware globally, you can specify individual routes to exclude in the VerifyCsrfToken middleware.
For Laravel 11 apps, here’s how you can exclude specific routes by passing their URIs to the validateCsrfTokens method in your application's bootstrap/app.php file.
->withMiddleware(function (Middleware $middleware) {
$middleware->validateCsrfTokens(except: [
'stripe/*',
'http://example.com/foo/bar',
'http://example.com/foo/*',
]);
})
For Laravel 10 or earlier, you can except some URLs from CSRF by editing the $except array in app/Http/Middleware/VerifyCsrfToken.php
namespace App\Http\Middleware;
use Illuminate\Foundation\Http\Middleware\VerifyCsrfToken as Middleware;
class VerifyCsrfToken extends Middleware
{
/**
* The URIs that should be excluded from CSRF verification.
*
* @var array<int, string>
*/
protected $except = [
'/some-page',
'/api/endpoint',
];
}
Best Practices to Avoid the “419 Page Expired” Error
To reduce the frequency of 419 errors, consider the following best practices:
- Prompt Users with Expiring Sessions: If you have forms where users tend to take a long time (e.g., filling out lengthy information), prompt them to save their progress or refresh the page to prevent token expiration.
- Enable Automatic CSRF Token Refresh: Consider using JavaScript to refresh the CSRF token in the background if users keep the page open for long periods.
- Log CSRF-Related Errors: Set up logs specifically for CSRF token issues. This can help identify recurring errors related to specific user actions or routes.
Now that you know what’s behind the “419 Page Expired” error, you’re prepared to tackle it head-on.
Keep building! 🚀