Mastering Laravel Collections
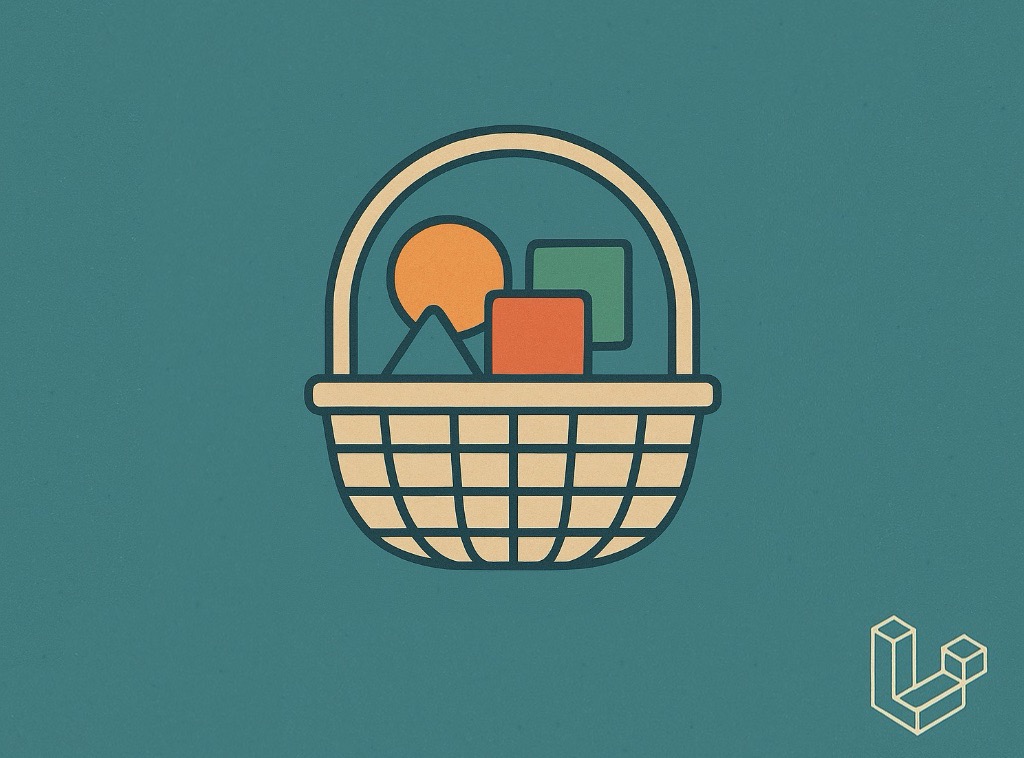
Laravel’s Collection class is one of the most powerful and expressive features of the framework. While it often flies under the radar for newcomers, mastering collections can significantly simplify your code, increase readability, and give you more control over your data transformations.
In this article, we’ll explore what collections are, when to use them, and how to truly master them with practical examples and tips.
Let's dive deeper 👇
What Are Laravel Collections?
A Collection is essentially a wrapper around arrays that provides a fluent, chainable interface for working with data. It’s an instance of the Illuminate\Support\Collection
class and comes with dozens of helpful methods for filtering, transforming, and aggregating data.
You typically interact with collections when working with Eloquent or manually converting arrays:
$collection = collect([1, 2, 3]);
Why Use Collections?
-
Readable and expressive code: Collections let you chain multiple operations in a way that’s clean and easy to follow. You end up with less boilerplate and more intent-driven code.
-
Immutable by design: Most collection methods return a new instance instead of modifying the original, which helps avoid unexpected side effects and makes debugging easier.
-
Consistent API: Whether your data comes from a database, an API, or a config file, the collection methods work the same way, no need to switch mental models.
-
Powerful utilities: Collections come with dozens of useful methods for tasks like grouping, filtering, mapping, flattening, and sorting, tools that would otherwise require a lot more manual code.
Core Collection Methods You Should Know
Here are the most commonly used collection methods to get comfortable with:
1. all()
Returns the underlying array from the collection.
collect([1, 2, 3])->all();
// [1, 2, 3]
2. each()
Loops through each item in the collection and runs the given callback on it.
collect([1, 2, 3])->each(fn($item) => logger($item));
3. chunk()
Breaks the collection into smaller collections of a given size.
collect([1, 2, 3, 4, 5])->chunk(2);
// [[1, 2], [3, 4], [5]]
4. collapse()
Flattens an array of arrays into a single-level collection.
collect([[1, 2], [3, 4]])->collapse();
// [1, 2, 3, 4]
5. contains()
/ containsStrict()
Checks whether the collection has a given value. The strict version checks with ===
.
collect([1, 2, 3])->contains(2); // true
collect([1, 2, '3'])->containsStrict(3); // false
6. dot()
Converts a nested associative array into a flat array using dot notation for keys.
collect(['user' => ['name' => 'Ahmad']])->dot();
// ['user.name' => 'Ahmad']
7. flatten()
Reduces a multi-dimensional collection into a flat, single-dimensional one.
collect([1, [2, [3]]])->flatten();
// [1, 2, [3]]
8. join()
Combines all items into a single string with custom separators.
collect(['a', 'b', 'c'])->join(', ', ' and ');
// 'a, b and c'
9. map()
Applies a transformation to each item and returns a new collection.
collect([1, 2, 3])->map(fn($i) => $i * 2);
// [2, 4, 6]
10. reduce()
Combines all items into a single value using a callback function.
collect([1, 2, 3])->reduce(fn($carry, $item) => $carry + $item);
// 6
11. filter()
Keeps only the items that pass the given condition.
collect([1, 2, 3])->filter(fn($i) => $i > 1);
// [2, 3]
12. pluck()
Extracts the values for a specific key from each item in the collection.
collect([['name' => 'A'], ['name' => 'B']])->pluck('name');
// ['A', 'B']
13. search()
Finds the index/key of the first item that matches the given value.
collect(['a', 'b', 'c'])->search('b');
// 1
14. take()
Returns a limited number of items from the beginning of the collection.
collect([1, 2, 3])->take(2);
// [1, 2]
15. groupBy()
Groups the collection’s items by a given key or result of a callback.
collect([
['type' => 'fruit', 'name' => 'apple'],
['type' => 'vegetable', 'name' => 'carrot'],
])->groupBy('type');
// ['fruit' => [...], 'vegetable' => [...]]
16. when()
Runs the callback only if the given condition is true.
$addMore = true;
collect([1, 2])->when($addMore, fn($c) => $c->push(3));
// [1, 2, 3]
17. tap()
Allows you to perform side effects on the collection without changing it.
collect([1, 2, 3])->tap(fn($c) => logger($c));
// [1, 2, 3]
18. partition()
Splits the collection into two based on a condition, one that passes and one that doesn't.
collect([1, 2, 3, 4])->partition(fn($i) => $i % 2 === 0);
// [[2, 4], [1, 3]]
19. flatMap()
Maps each item and then flattens the result by one level.
collect([1, 2])->flatMap(fn($i) => [$i, $i * 10]);
// [1, 10, 2, 20]
20. only()
Returns only the specified keys from the collection.
collect(['a' => 1, 'b' => 2])->only(['a']);
// ['a' => 1]
21. except()
Removes the specified keys from the collection.
collect(['a' => 1, 'b' => 2])->except(['b']);
// ['a' => 1]
Practical Collection Examples
Below are practical, real-world examples that save you time, eliminate repetitive boilerplate, and keep your code clean, expressive, and easy to maintain.
1. Flattening and Uniquing Nested Tags
Use case: Get a unique list of all tags used in blog posts.
$allTags = collect($posts)
->pluck('tags') // returns a collection of arrays
->flatten()
->unique()
->values();
2. Summing Values Conditionally
Use case: Calculate income from only completed orders.
$totalRevenue = collect($orders)
->where('status', 'paid')
->sum('amount');
3. Chaining filter
, map
, and reduce
Use case: Compute cart total for in-stock items only.
$totalCost = collect($cartItems)
->filter(fn($item) => $item['in_stock'])
->map(fn($item) => $item['price'] * $item['quantity'])
->reduce(fn($carry, $cost) => $carry + $cost, 0);
4. Filtering Out Null or Empty Values
Use case: Remove null
, false
, ''
, []
, etc. from an input array.
$cleaned = collect($input)->filter();
5. Generating a Keyed List for Dropdowns
Use case: Prepare an associative array for <select>
options.
$dropdown = collect($categories)
->sortBy('name')
->pluck('name', 'id');
6. Build a Full Name List from User Records
Use case: Useful when displaying lists of users with their full names.
$fullNames = collect($users)
->map(fn($user) => $user['first_name'] . ' ' . $user['last_name']);
7. Filter and Paginate a Collection Manually
Use case: When you're working outside of Eloquent scope or need manual pagination logic.
$page = 2;
$perPage = 10;
$paged = collect($items)
->forPage($page, $perPage)
->values();
8. Convert a List of Emails to Lowercase and Unique
Use case: Prevents duplicate emails due to casing issues.
$emails = collect($subscribers)
->pluck('email')
->map(fn($email) => strtolower($email))
->unique()
->values();
9. Combine Two Fields into One Key
Use case: Useful for quick lookups when you need compound keys.
$lookup = collect($users)
->mapWithKeys(fn($user) => [
$user['id'] . ':' . $user['email'] => $user
]);
That was a quick look at some of the most useful Laravel collection methods. You probably won’t need all of them every day, but knowing what’s available can save you time and help keep your code readable. The more you work with collections, the more second nature they become.
Keep building great stuff! 🚀