12 Top Security Best Practices for Your Laravel Application
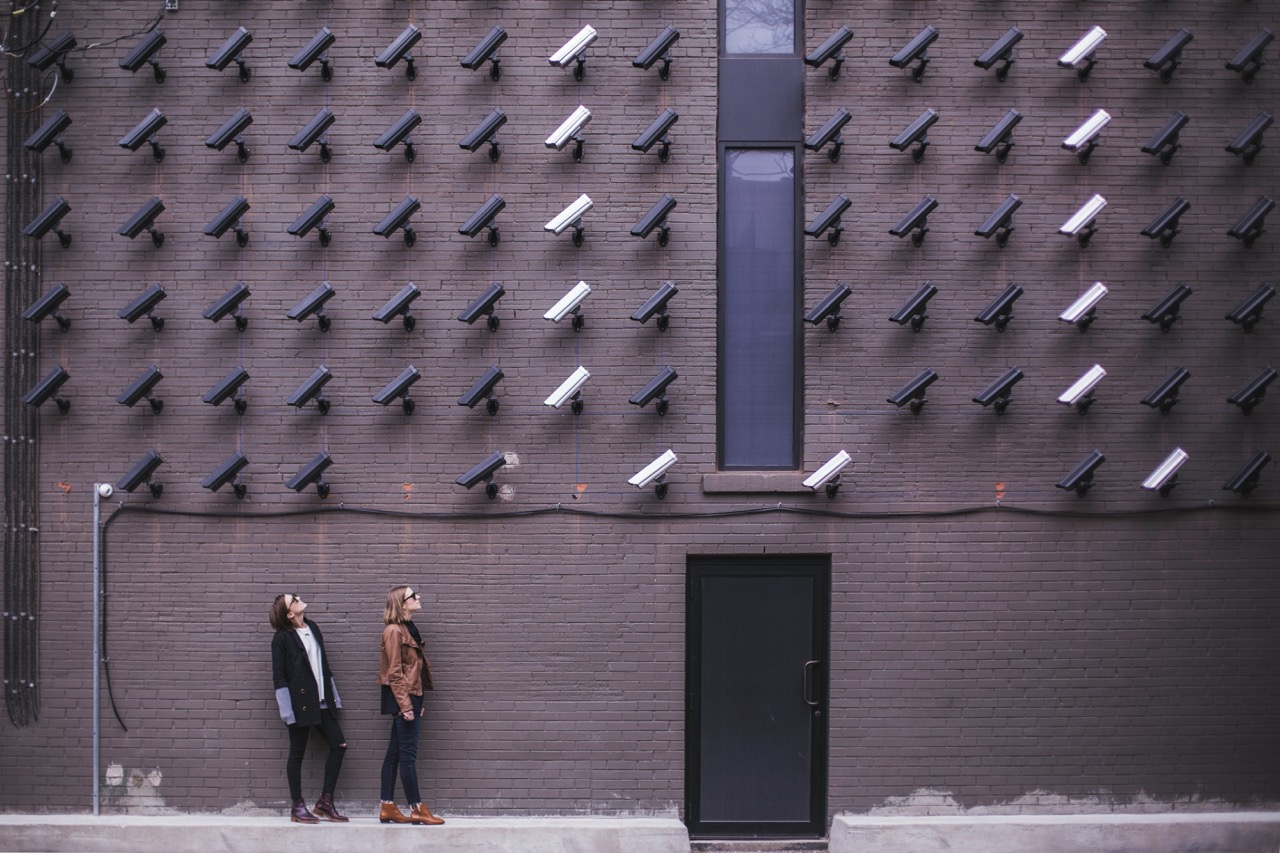
In today’s world, where cyber threats are more pervasive than ever, security is not an optional consideration but an absolute necessity. The stakes are high: a single security lapse could compromise sensitive data and, in the worst-case scenario, jeopardize your entire business. As digital attacks become increasingly sophisticated, securing your Laravel application is crucial to protecting your business and maintaining user trust.
So let's dive in.
1. Keep Laravel and Dependencies Updated
One of the simplest and most effective ways to secure your Laravel application is to keep both the Laravel framework and its dependencies up to date. Laravel frequently releases security patches, and using outdated versions can leave your application vulnerable to known exploits.
- Run composer update regularly.
- Keep an eye on Laravel’s official blog or GitHub repository for updates.
- Enable automatic security updates for dependencies using services like Dependabot (renamed to Github security).
2. Enforce HTTPS
Use HTTPS to ensure all data transmitted between your users and your application is encrypted. To enforce HTTPS, make sure to set the following in your AppServiceProvider
public function boot()
{
if (config('app.env') === 'production') {
\URL::forceScheme('https');
}
}
Additionally, ensure that you have a valid SSL certificate installed on your server. Use Let's Encrypt to get a free SSL certificate if needed.
3. Secure Authentication
Laravel’s built-in authentication system provides a solid foundation, but there are additional measures you can take to ensure a higher level of security:
-
Use strong passwords: Require your users to use strong passwords by using Laravel’s Password::defaults() method to enforce stricter password policies.
-
Implement two-factor authentication (2FA): You can integrate packages like Laravel Jetstream to enable 2FA in your app.
-
Account lockout after failed attempts: Implement a system that locks out accounts after a certain number of failed login attempts using Laravel’s ThrottleRequests middleware.
use Illuminate\Support\Facades\Route; use App\Http\Controllers\Auth\LoginController; Route::post('/login', [LoginController::class, 'login']) ->middleware('throttle:5,1'); // Allow 5 login attempts per minute
4. Sanitize and Validate Input
One of the fundamental principles in web development is to never trust user input. Whether it's data coming from a form, URL parameters, or file uploads, you should always treat it as untrusted and potentially malicious. Users may inadvertently—or intentionally—submit harmful data, which could lead to severe security vulnerabilities, such as SQL injection, Cross-Site Scripting (XSS), and remote code execution.
$request->validate([
'email' => 'required|email',
'password' => 'required|min:8',
]);
Also, consider usiny HTMLPurifier to clean and filter any HTML content that users may be able to submit. This helps prevent XSS (Cross-Site Scripting) attacks.
5. Preventing Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) is a common security vulnerability where attackers inject malicious scripts into web pages viewed by other users. If your Laravel application is not properly secured against XSS, attackers could exploit this to steal user data, hijack sessions, or perform other harmful actions.
Fortunately, Laravel provides several built-in features to help prevent XSS attacks and ensure your application remains secure.
- Automatic Output Escaping
Laravel's Blade templating engine automatically escapes all data output to prevent XSS. When you use the {{ }} syntax to display data, Blade will escape any special characters, ensuring that injected scripts will not be executed in the browser.
{{ $userInput }}
This will automatically convert characters like <, >, and & into their HTML entity equivalents, effectively neutralizing any potentially harmful scripts.
- Escaping Raw Output
In cases where you want to display raw HTML, you can use the {!! !!} syntax. However, this should be used cautiously, as it does not provide XSS protection. Only use it when you are certain the output is safe.
{!! $trustedHtml !!}
Important: To ensure safety, always sanitize the data before outputting it as raw HTML.
- Using e() Helper for Manual Escaping
If you're working outside of Blade templates or need to manually escape output, you can use Laravel's e() helper function. It works similarly to Blade’s automatic escaping.
echo e($userInput);
This ensures any user-generated content is properly escaped and safe for display.
6. Protect Against CSRF Attacks
Cross-Site Request Forgery (CSRF) is a type of attack where malicious sites trick users into executing unwanted actions on your application. Laravel includes CSRF protection out of the box. Always ensure you’re using the @csrf Blade directive in your forms:
<form method="POST" action="/submit">
@csrf
<!-- form fields -->
</form>
Laravel’s CSRF token verification is automatically applied to all POST, PUT, PATCH, and DELETE requests.
7. Secure Your Routes and Controllers
Limit access to certain routes and controllers by using Laravel’s built-in authorization features. Use middleware to protect routes based on the user’s role or permissions. For example:
Route::group(['middleware' => ['auth', 'role:admin']], function () {
Route::get('/admin', [AdminController::class, 'index']);
});
You can also define more fine-grained policies using Laravel’s authorization gates and policies.
8. Avoid Query Vulnerabilities with Eloquent ORM
Laravel’s Eloquent ORM helps protect against SQL injection attacks by using prepared statements for database queries. Avoid raw SQL queries unless absolutely necessary, and when you do use them, make sure to use bindings to protect against injection:
DB::select('SELECT * FROM users WHERE email = ?', [$email]);
Using Eloquent’s query builder is always a safer option:
$user = User::where('email', $email)->first();
9. Encrypt Sensitive Data
Encrypt sensitive user data, especially things like passwords, personal information, or API tokens. Laravel makes this easy by providing the Crypt facade for encryption.
For example, to encrypt a piece of data:
$encrypted = Crypt::encrypt($data);
And to decrypt it:
$decrypted = Crypt::decrypt($encryptedData);
For user passwords, always use Laravel's built-in hashing functions:
use Illuminate\Support\Facades\Hash;
Hash::make('password');
10. Limit the Exposure of Debugging Information
Never leave your application in debug mode in a production environment, as it can expose sensitive information such as database credentials, stack traces, and environment variables.
Make sure the APP_DEBUG setting is set to false in your .env file for production:
APP_DEBUG=false
Also, ensure that you are logging errors correctly and securely.
11. Avoid Using $request->all()
One common mistake when handling user input in Laravel is relying on $request->all() in store or update methods. This practice can introduce security vulnerabilities by allowing unfiltered input from the frontend or an API, potentially exposing sensitive fields to manipulation.
For example, if your database has a field like is_admin and it's fillable in your Eloquent model, a malicious user could modify the HTML or API request and include this field in the form data. This could elevate their privileges by changing a simple boolean value.
Instead of using $request->all(), adopt a safer approach by using Form Request classes for validation. After validation, you can retrieve the validated input with $request->validated(). Additionally, use $request->only() or $request->except() to explicitly define which fields you expect from the form, ensuring that no extra or harmful data is passed to your database.
namespace App\Http\Controllers;
use App\Http\Requests\StoreUserRequest;
use App\Models\User;
class UserController extends Controller
{
public function store(StoreUserRequest $request)
{
// Using $request->validated() to get only the validated data.
$validatedData = $request->validated();
// You can also explicitly specify which fields to accept.
$data = $request->only(['name', 'email', 'password']);
// Alternatively, if you want to exclude specific fields:
// $data = $request->except(['password_confirmation']);
// Create a new user with the validated data.
$user = User::create([
'name' => $data['name'],
'email' => $data['email'],
'password' => bcrypt($data['password']),
]);
return redirect()->route('users.index')->with('success', 'User created successfully.');
}
}
This practice greatly reduces the risk of unauthorized input making its way into your application, keeping your data safe from potential manipulation or attacks.
12. Regular Backups and Monitoring
Regularly backup your Laravel application and databases. You can use a package like Spatie Laravel Backup to automate backups.
Also, implement logging and monitoring tools to track any suspicious activities or potential security breaches. Laravel supports a variety of logging drivers, including Syslog, Slack, and more.
Important: Don't log senstive data!
When logging information in your Laravel application, it’s crucial to sanitize sensitive data like credentials, tokens, or personally identifiable information (PII) before storing them in the logging system. Logging sensitive data without sanitization can expose your application to security risks, especially if logs are accessed by unauthorized individuals or shared with third-party systems.
One of the best practices when logging sensitive data is to implement a whitelist approach, where only explicitly approved fields are logged. This ensures that only non-sensitive information is stored, and all other fields, especially those containing credentials or PII, are either omitted or sanitized. Instead of blacklisting specific fields (which can be error-prone and might miss some sensitive data), a whitelist approach ensures that only safe, pre-approved data is logged.
For example, when logging user-related actions, you could whitelist fields such as the user's email or ID, while automatically sanitizing or excluding sensitive fields like passwords, tokens, or personal addresses. Any field not included in the whitelist should be treated as sensitive and either masked or excluded entirely from the logs.
$whitelistedFields = ['email', 'action'];
$dataToLog = array_intersect_key($request->all(), array_flip($whitelistedFields));
// Log only the whitelisted data
Log::info('User action logged', $dataToLog);
Bonus: Secure Job Payloads with Encryption
When you dispatch a job in Laravel, the job's payload gets stored in a backend like Redis, a database, or whatever storage system you've configured via the QUEUE_CONNECTION environment variable.
Since job payloads can contain sensitive data, there's a risk that someone with access to your storage might misuse that information.
Laravel offers a simple way to safeguard these payloads by using the Illuminate\Contracts\Queue\ShouldBeEncrypted contract. This contract ensures the payload is automatically encrypted when it's stored. Laravel uses the application key in the (.env) file to encrypt & later decrypt the payload, so make sure that this is stored securely.
Here’s an example of how to implement encrypted job payloads:
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Contracts\Queue\ShouldBeEncrypted;
class ProcessOrder implements ShouldQueue, ShouldBeEncrypted
{
// Handle the job's logic here
}
Securing your Laravel application is a continuous process that requires a proactive approach. By following these best practices, you can protect your app from many common vulnerabilities and attacks.
Remember, security isn't just about adding features or tools; it's about cultivating a mindset that prioritizes protection at every stage of development. From authentication systems to database queries, each layer of your application should be scrutinized for potential risks.
By integrating these strategies, you'll create a Laravel app that is not only high-performing but also resilient against the growing landscape of cyber threats.
Keep building! 🚀