5 Practical Use Cases for Laravel defer() Function
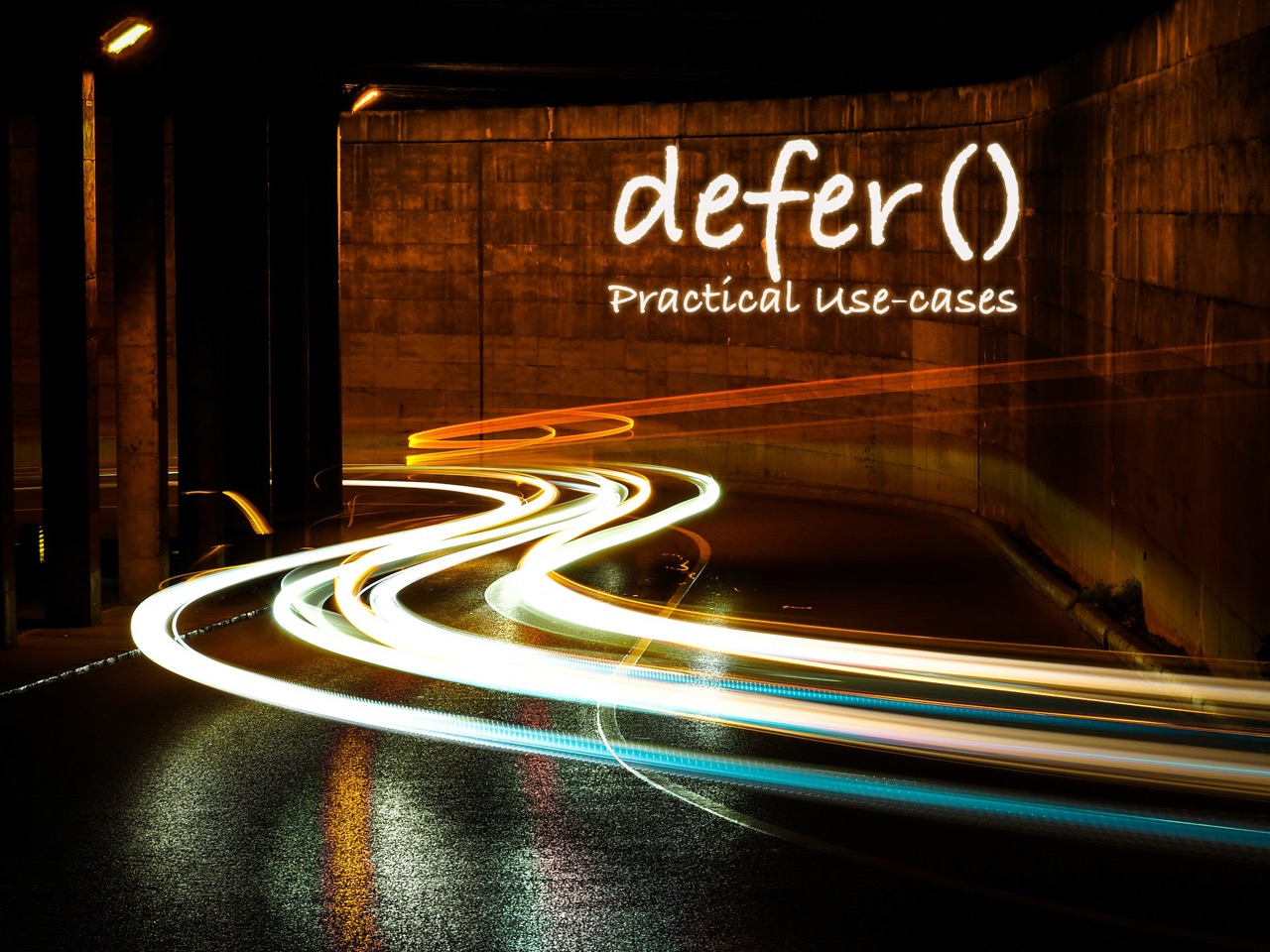
In modern web development, speed and responsiveness are king. A slow-loading website or application can be the difference between user satisfaction and frustration. Laravel’s defer() function is a new addition designed to address this by allowing you to perform background tasks effortlessly without affecting response times.
In this article, we’ll explore why defer() is a fantastic addition to Laravel and dive into practical use cases, complete with code examples.
Let’s get started.
Why Use Laravel's defer() Function?
Traditionally, developers have relied on queued jobs or background processes to handle tasks that don’t need to be completed immediately. While effective, these approaches come with overhead: setting up queue workers, configuring job classes, and managing the infrastructure, etc.
The defer() function simplifies this process. Here’s why it’s awesome:
1. Simplicity of Use
Using defer() is straightforward. There’s no need to set up workers or configure queue drivers. Just wrap the task in a closure, and Laravel will ensure it runs after the response is sent.
Example:
use Illuminate\Support\Facades\Response;
Route::post('/process', function () {
defer(function () {
// Task to run after the response
logger('Deferred task executed.');
});
return Response::json(['message' => 'Task is being processed!']);
});
2. Centralized Background Functionality
Instead of scattering background jobs across different job classes or firing events, defer() lets you keep related deferred logic in the same place as the main request-handling logic. This improves code readability and maintainability.
3. Improved User Experience
Since the tasks run after the response is sent, the user gets a faster experience while the server continues handling non-urgent operations in the background.
Practical Use Cases for defer()
Let’s explore some real-world scenarios where defer() can shine. 👇
1. Third-Party API Communications
When your app needs to send data to an external service, such as an analytics platform, it can delay the response. Using defer(), you can offload this task.
Code Example:
use Illuminate\Support\Facades\Http;
Route::post('/purchase', function () {
defer(function () {
Http::post('https://analytics.example.com/log', [
'event' => 'purchase',
'timestamp' => now(),
]);
});
return response()->json(['message' => 'Purchase successful!']);
});
Benefit: The user immediately sees a confirmation while the analytics data is processed in the background.
2. Sending Emails
Sending emails can be a time-consuming operation, especially if it involves attachments. With defer(), you can process emails after the response.
Code Example:
use Illuminate\Support\Facades\Mail;
Route::post('/register', function () {
defer(function () {
Mail::to('[email protected]')->send(new WelcomeEmail());
});
return response()->json(['message' => 'Welcome email is on its way!']);
});
Benefit: Users don’t have to wait for the email to be sent before they see the registration success message.
3. Cleaning Up Temporary Files After Download
When generating temporary files for downloads or processing, cleaning them up after the response ensures your server stays clean without impacting the user experience.
Code Example:
use Illuminate\Support\Facades\Storage;
Route::get('/download', function () {
$filePath = 'temporary/report.pdf';
// Generate the file
Storage::put($filePath, 'Generated report content');
defer(function () use ($filePath) {
Storage::delete($filePath);
});
return Storage::download($filePath);
});
Benefit: Users download their files while the server cleans up temporary files in the background.
4. Webhook Handling
Responding quickly to webhooks from external services is crucial. With defer(), you can send an acknowledgment response and process the payload later.
Code Example:
Route::post('/webhook', function () {
defer(function () {
// Process the webhook payload
logger('Webhook processed.');
});
return response()->json(['status' => 'success']);
});
5. Complex Calculations
For CPU-intensive operations, such as generating reports or performing calculations, defer() allows you to process these in the background.
Code Example:
Route::get('/generate-report', function () {
defer(function () {
// Simulate a heavy calculation
sleep(5);
logger('Report generation complete.');
});
return response()->json(['message' => 'Report generation started!']);
});
Benefit: Users don’t experience delays while the server completes resource-intensive tasks.
Conclusion
Laravel’s defer() function brings a much-needed improvement to handling background tasks. It’s simple to use, keeps your deferred logic in one place, and ensures a better user experience by running non-urgent tasks after the response.
If you haven’t tried it yet, now is the perfect time to start including it into your projects!
Keep building! 🤘