How to Generate Documentation for Your Laravel Project
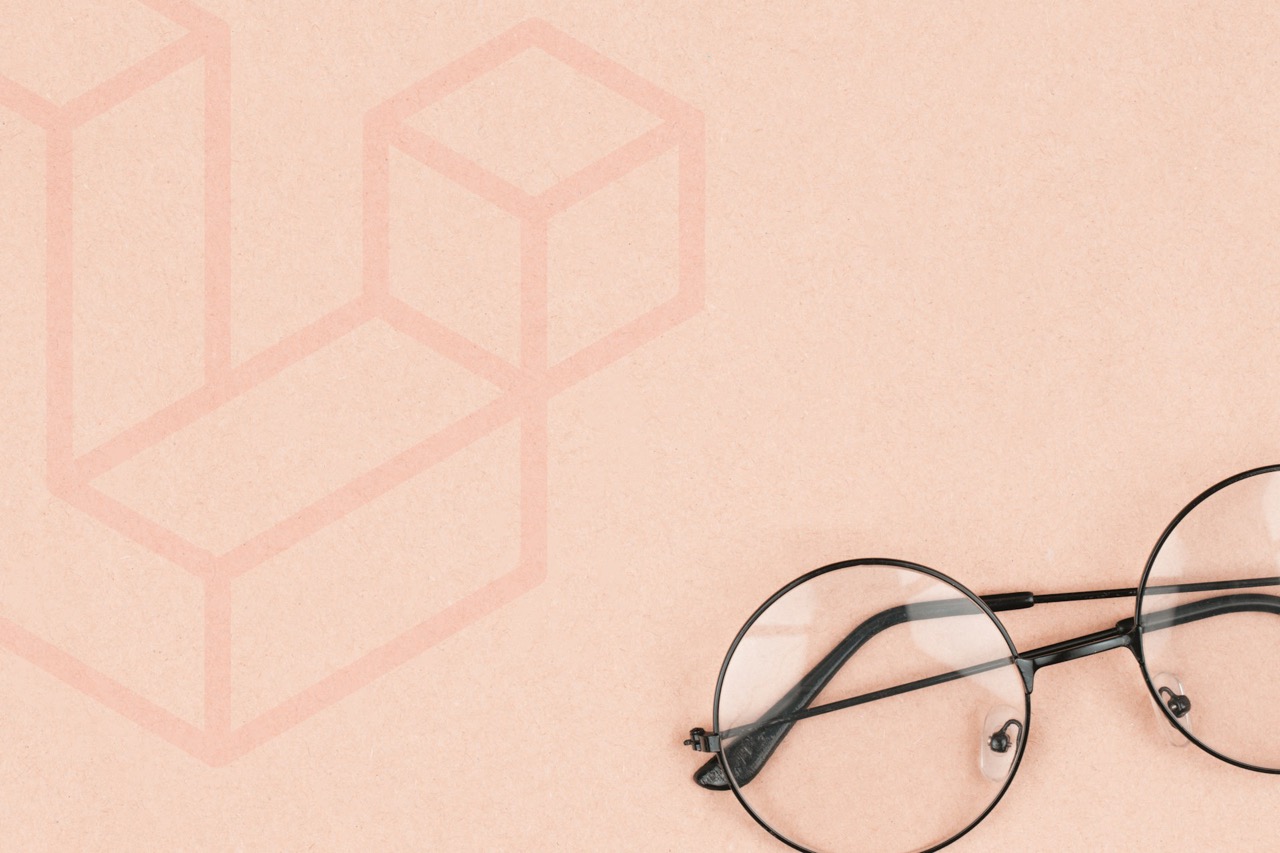
In software development, writing code is just the beginning. One thing that truly sets exceptional projects apart is the quality of their documentation.
Clear, well-organized documentation not only enhances collaboration among developers/project stakeholders, but also provides valuable guidance for end users, making your product more accessible and easier to use.
For Laravel projects, whether you're building a simple app, an API, or a user-facing product, great documentation is essential, and luckily, there are plenty of tools that can help you with that.
In this article, we’ll explore how to create high-quality documentation for your Laravel project, using tools like Scribe, PHPDoc, Jigsaw, and Docusaurus to cater to both developers and product users alike.
Let's dive in. 👇
Why Good Documentation Matters
Good documentation helps:
- Onboarding new developers: It provides clear guidance for setting up and understanding the project.
- Onboarding product users: Well-written user guides and tutorials help users get the most out of your product without confusion.
- Maintenance: Proper documentation helps you and others understand your project's structure, making debugging and updates easier.
- Collaboration: Clear docs promote efficient collaboration, whether on open-source or team projects.
- Reducing support tickets: This is a BIG one. Comprehensive, easy-to-follow documentation enables users to resolve common issues on their own, lowering the number of support requests.
1. Generating API Documentation with Scribe
For projects that expose APIs, auto-generating API documentation would save you a lot of trouble. Scribe is a Laravel-specific package that generates API documentation based on your routes and annotations.
Installing and Using Scribe
Install it via Composer:
composer require knuckleswtf/scribe
Publish the config file and generate your docs:
php artisan vendor:publish --tag=scribe-config
Generate your docs:
php artisan scribe:generate
Scribe scans your routes and produces API documentation in Markdown or HTML, which you can host as part of your project or online. Use annotations in your controllers to provide more details about the API:
Check the documenation of Scribe - which is done using Docusaurus by the way :) - for more on how to best use it.
2. Code-Level Documentation with PHPDoc and phpDocumentor
In addition to API documentation, you might need inline documentation to explain your code at the method and class level. This is where PHPDoc comes in. PHPDoc comments provide insights into what specific methods, properties, and classes do.
Using PHPDoc
Here’s an example of a well-documented method using PHPDoc:
You can generate structured documentation from PHPDoc comments using phpDocumentor
To install it using the PHAR archive, download the phar file from https://github.com/phpDocumentor/phpDocumentor/releases
Then you can run it using:
This will scan your code for PHPDoc comments and generate a full HTML documentation site.
3. Static Documentation Site with Jigsaw
For custom documentation that needs to be more extensive (e.g., explaining business logic, installation steps, configuration, etc.), using a static site generator like Jigsaw is a great choice.
Setting Up Jigsaw for Documentation
Jigsaw is a Laravel-based static site generator that is simple to use for documentation. Install Jigsaw globally via Composer:
Then, initialize a documentation site:
Jigsaw creates a skeleton project with folders for writing Markdown content. Once you’ve written your docs, you can build the site using:
This generates static HTML files that can be hosted anywhere, like GitHub Pages or Netlify.
4. Creating a Full Documentation Website with Docusaurus
If you want a more polished, production-ready documentation site with advanced features like versioning, search, and beautiful themes, Docusaurus is a perfect option. Docusaurus is a React-based static site generator built by Facebook, ideal for projects that need comprehensive, scalable docs.
Why Use Docusaurus?
Docusaurus offers:
- Markdown support: Write your docs in Markdown, just like Jigsaw.
- Versioning: Support different versions of your documentation, useful for projects with multiple releases.
- Search: Out-of-the-box search functionality for easy navigation.
- Internationalization: Easily serve your documentation in different languages.
- React components: If you need interactivity or custom UI elements.
Setting Up Docusaurus
-
Install Docusaurus: To install Docusaurus, run the following commands:
-
Configure Documentation: The docs directory will contain your Markdown files for documentation. You can start by editing the example docs in docs/intro.md or add new files.
-
Run Development Server: To see your documentation live, use the development server:
Docusaurus will generate a local server where you can preview your documentation site.
-
Build the Documentation Site: When you're ready to build the site, use the following command to generate static files:
-
Versioning: Docusaurus supports versioning for your documentation. After releasing a new version of your Laravel project, run the versioning command:
This creates a snapshot of your current docs, allowing you to maintain separate documentation for different versions of your project.
Deploying Docusaurus
You can deploy Docusaurus on any static site hosting platform like GitHub Pages or Netlify or Vercel. For GitHub Pages:
npm run deploy
This command will automatically push your static files to the gh-pages branch of your GitHub repo and make your docs site live.
Deploying Docusaurus docs within a Laravel app
You can host your Docusaurus documentation inside your Laravel project if you prefer. In fact, this is how I host the documenation of SaaSykit.
I host the docusaurus project (where the markdown documentation is) in a separate Github repository. On my local machine I have this repository located in the same directory as SaaSykit's website project.
Then in the "package.json" inside the docusaurus project I definded a new command -> "build-in-saasykit-website" (see below)
// ....
"scripts": {
"docusaurus": "docusaurus",
"start": "docusaurus start",
"build": "docusaurus build",
"build-in-saasykit-website": "docusaurus build --out-dir ../saasykit-website/public/docs",
"swizzle": "docusaurus swizzle",
"deploy": "docusaurus deploy",
"clear": "docusaurus clear",
"serve": "docusaurus serve",
"write-translations": "docusaurus write-translations",
"write-heading-ids": "docusaurus write-heading-ids"
},
// ....
As you might have guessed, this command generates the documenation statis files inside the "public/docs" directory within SaaSykit's website (a Laravel application), using the relative path of the project.
npm run build-in-saasykit-website
As these files are inside the "public" directory, users can access them on the web.
Important: make sure that your web server is properly configured to serve static files from the public directory in your Laravel app (I had to do that with Caddy).
5. README and Markdown Files
No matter which tool you use for detailed docs, always maintain a high-quality README.md file within your project's repository. This should include:
- Project Overview: A brief summary of what your project does.
- Installation Instructions: How to set up and run the project.
- Usage: Examples or key commands.
- Contribution Guide: Instructions for contributing to the project if it's an open source project.
A basic example might look like this:
Conclusion
Documenting your Laravel project doesn't have to be a burden. With the right tools, like Scribe, PHPDoc, Jigsaw, and Docusaurus, you can create clear, organized documentation that serves both your development team and your product users.
By investing time in writing & automating the generation of high-quality documentation, you’re not only improving collaboration and easing maintenance but also enhancing the user experience and reducing support requests, saving your future self much time and effort. Good docs aren't just an add-on, they’re a foundation for long-term success in any project.
So keep writing great documentation ... and who knows, maybe one day your docs will be so good that no one will even need to ask you for help anymore. Just imagine all the free time you'll have! 😃
Keep building! 🤘