How to Not Turn Your Laravel App into a Circus
By
Amas
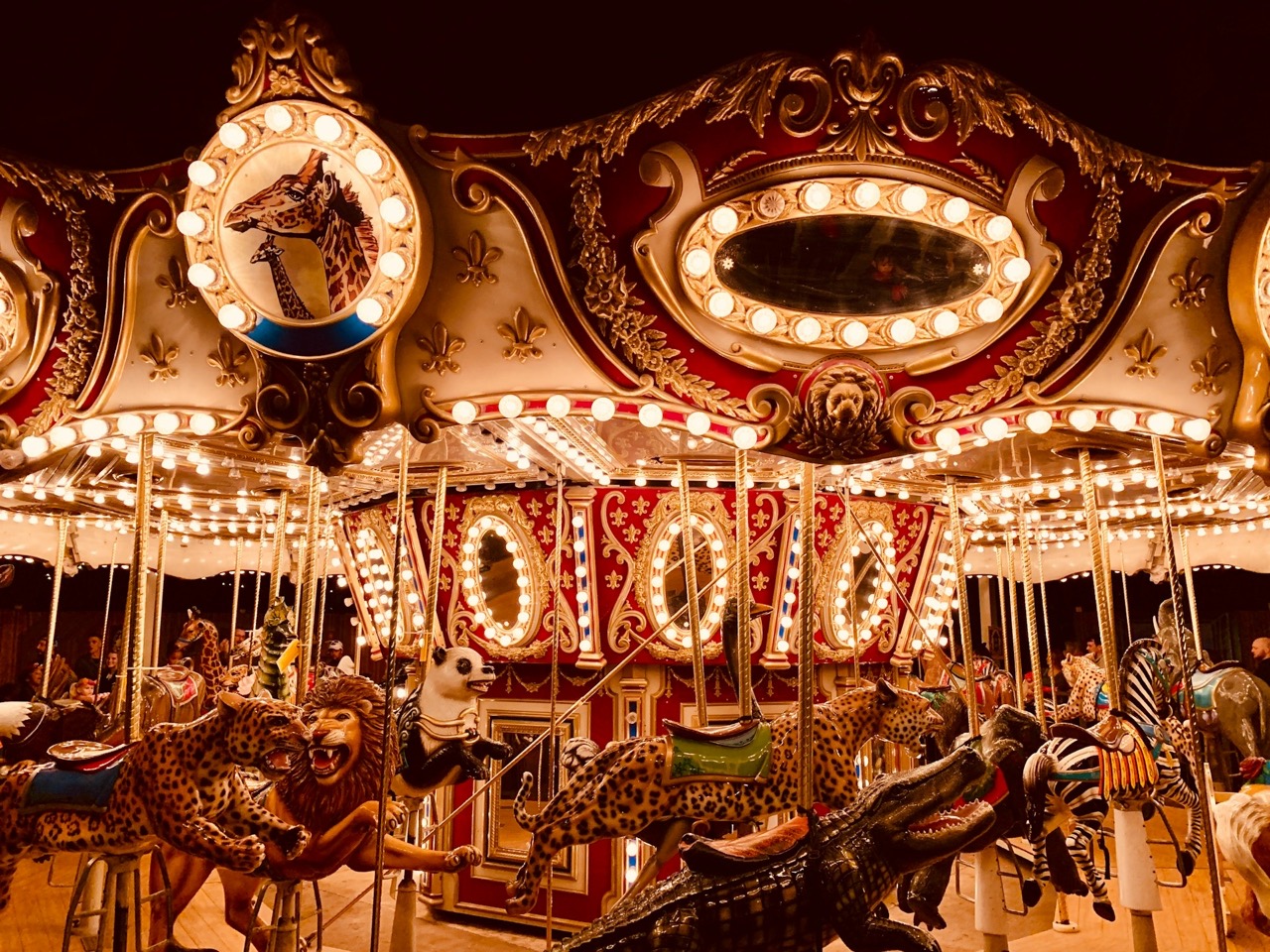
Laravel is a powerful and elegant PHP framework that can help you build robust and scalable web applications. However, like any tool, it’s only as good as the developer using it. Poor practices can quickly turn your elegant codebase into an unmanageable circus. In this article, we’ll explore common pitfalls and how to avoid them, ensuring your Laravel application remains clean, efficient, and maintainable.
1. Ignoring Best Practices
Laravel has well-established conventions and best practices, which are there for a reason. Ignoring them is like walking a tightrope without a safety net. Following these practices helps keep your codebase consistent, making it easier for you and others to understand and maintain.
Example: Using route closures for complex logic instead of controllers.
Solution: Adhere to Laravel’s conventions, like using controllers for logic and routes for defining endpoints.
2. Overcomplicating the Architecture
It’s easy to over-engineer your Laravel application, especially with the abundance of features Laravel provides. However, adding unnecessary layers of abstraction can turn your app into a maze of confusion.
Example: Implementing a repository pattern for every model, even when not needed.
Solution: Stick to simplicity and only introduce complexity when there’s a clear benefit.
3. Messy Migrations
Database migrations are a powerful feature of Laravel, but treating them carelessly can lead to big chaos. Poorly planned migrations, or worse, editing existing ones, can make your database a house of mirrors.
Example: Altering a migration that has already been run in production.
Solution: Never modify existing migrations. Instead, create new migrations for changes, ensuring a clear history of your database evolution.
4. Hardcoding Configurations
Hardcoding configurations is like trying to tame a wild animal; it’s unpredictable and dangerous. This practice leads to inflexible and unscalable applications that are difficult to manage.
Example: Hardcoding API keys or environment-specific URLs in your controllers.
Solution: Use Laravel’s configuration files and environment variables (.env) to manage your app’s configurations securely and flexibly.
5. Spaghetti Code in Controllers
Controllers should be the ringmasters of your application, orchestrating the flow of data and logic. However, cramming them with business logic and database queries can turn them into an aweful sideshow.
Example: A controller method that handles validation, database queries, and business logic all in one place.
Solution: Utilize service classes, form requests, and repositories to keep your controllers lean and focused on directing traffic.
6. Neglecting Security Best Practices
Security is a critical part of any web application. Failing to follow security best practices can leave your Laravel app vulnerable to attacks.
Example: Not validating user input, leading to SQL injection vulnerabilities.
Solution: Always validate and sanitize user inputs, use Laravel’s built-in security features, and regularly update your dependencies.
7. Ignoring Testing
Skipping tests is like performing without a safety net. It’s tempting to bypass testing in the rush to deploy, but doing so can lead to disaster down the road.
Example: Deploying new features without any automated tests.
Solution: Implement unit and feature tests to ensure your application works as expected. Use Laravel’s testing suite to make this easier.
8. Overloading Blade Templates
Blade is Laravel’s powerful templating engine, but overloading your templates with complex logic is a common pitfall. This can lead to tangled, unmanageable views that are hard to debug.
Example: Writing complex @foreach loops and conditional logic directly in Blade templates.
Solution: Offload complex logic to view composers or dedicated helper classes, keeping your Blade templates clean and focused on presentation.
9. Not Leveraging Eloquent Properly
Eloquent, Laravel’s ORM, is a fantastic tool for interacting with your database. However, misusing it can quickly lead to performance issues and tangled relationships.
Example: Overusing eager loading or writing inefficient queries directly in your models.
Solution: Understand when to use eager loading versus lazy loading, and keep your Eloquent models lean by avoiding direct query logic in them.
10. Too Many Events
Events are a great way to decouple various parts of your application, but overusing them can turn your app into a chaotic circus of event handlers and listeners. Too many events can lead to performance bottlenecks and make your app difficult to debug.
Example: Firing events for every minor action, leading to a cascade of event listeners being triggered.
Solution: Use events wisely. Reserve them for significant actions where decoupling is truly beneficial, and avoid firing events for every trivial operation.
By avoiding these common pitfalls, you can keep your Laravel application running smoothly and avoid turning it into a chaotic circus. Remember, a clean, well-maintained codebase is the key to long-term success, both for your project and your sanity as a developer.
Keep building! 🚀