Optimizing Laravel Performance for High Traffic SaaS Applications
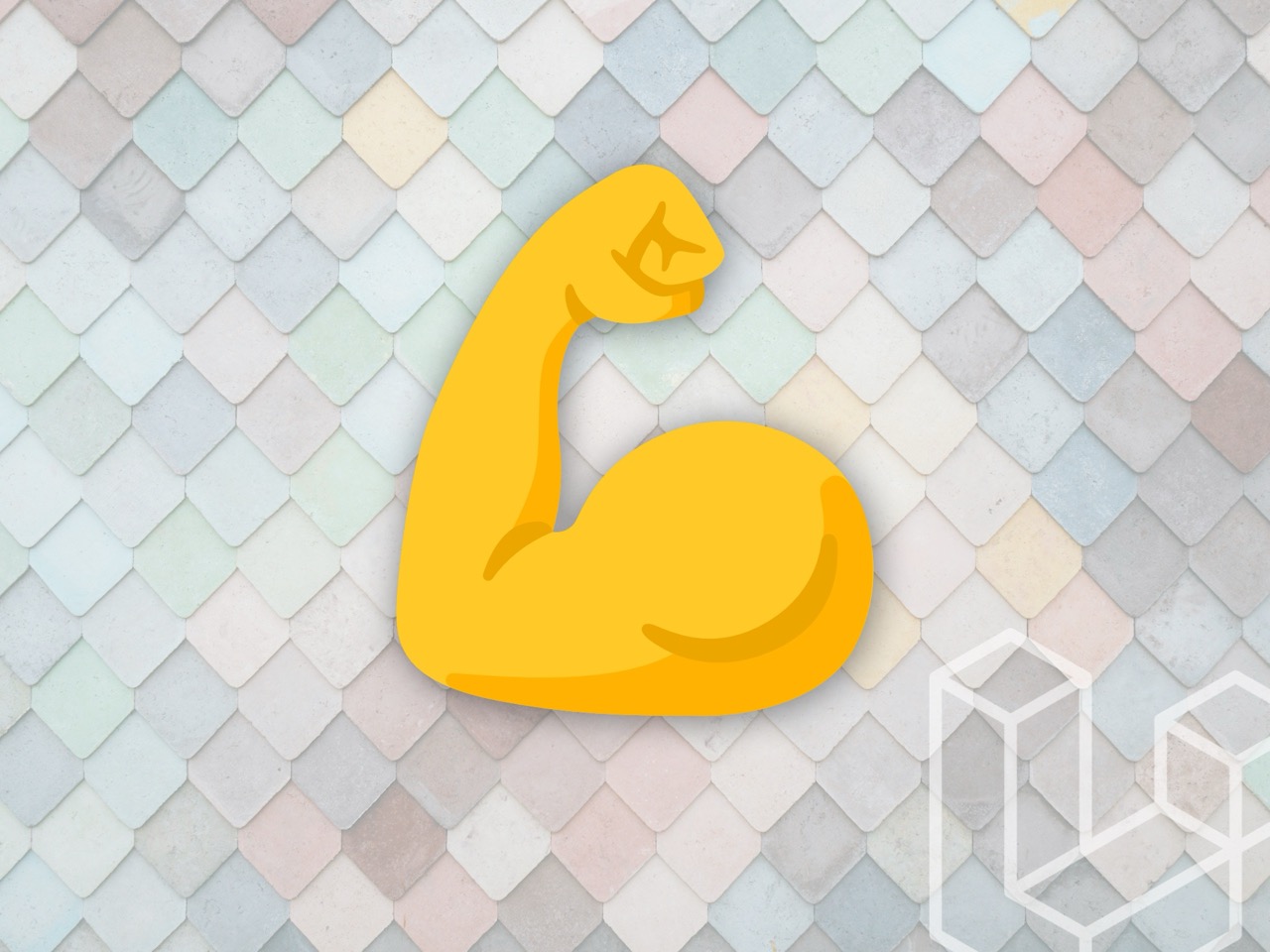
1. Optimize Your Database Queries
The database is often the first bottleneck in a high-traffic SaaS app. Laravel's Eloquent ORM makes querying a breeze, but it can lead to inefficient queries if not used carefully.
-
Use Eager Loading:
If you notice your app is making several database queries for related models (known as the N+1 query problem), eager loading can reduce the number of queries by loading related data upfront using with() in Eloquent.// Instead of: $users = User::all(); foreach ($users as $user) { echo $user->profile->bio; } // Use: $users = User::with('profile')->get(); foreach ($users as $user) { echo $user->profile->bio; }
-
Index Your Database:
Indexing columns that are frequently used in WHERE clauses or joins can drastically improve query performance. Use database indexing wisely by analyzing your query patterns. -
Use Queues for Heavy Queries:
For actions that don’t require an immediate response, such as report generation or data syncing, queue the task to be processed asynchronously using Laravel’s built-in queues. - Chunking Data for Large Data Sets:
When dealing with large datasets, retrieving all records in a single query can cause memory exhaustion or slow down your application significantly. Laravel provides a useful chunk() method to process large datasets in smaller, manageable chunks. This is particularly helpful when exporting data, running background tasks, or handling real-time updates.
// Processing users in chunks of 1000 records User::chunk(1000, function ($users) { foreach ($users as $user) { // Process each user } });
- Paginate for User-Facing Queries:
For user-facing queries that involve displaying large lists of data, always use pagination to avoid loading all results at once. Laravel's paginate() method is an excellent way to split results across multiple pages and minimize the load on your application.
// Paginate results, 15 items per page $users = User::paginate(15);
2. Caching is Key
Caching is essential for reducing server load and improving response times. Laravel provides a unified API for various caching systems, making it easy to cache complex data structures, views, and routes.
- Use Config and Route Caching:
Caching your configuration and routes can speed up your app's response times.
php artisan config:cache php artisan route:cache
- Cache Queries:
Frequently accessed data that doesn't change often (such as settings, product lists, or public content) should be cached. Use Laravel’s remember() method to store query results.$posts = Cache::remember('posts', 3600, function () { return Post::all(); });
- Use Redis or Memcached:
Redis and Memcached are two of the most popular in-memory caching systems that can significantly reduce the load on your database and improve response times by storing frequently accessed data in memory. This not only reduces the number of database queries but also allows your application to serve data much faster, especially for repetitive tasks like session management, API rate limiting, or retrieving frequently queried data.
Both Redis & Memcached are natively supported in Laravel, so you have no reason not to use them.
3. Use Queues and Job Prioritization
Laravel's queue system is a powerful tool for deferring time-consuming tasks like sending emails, processing files, or interacting with third-party services, allowing your application to offload these operations and improve performance for real-time user interactions. By pushing tasks into the background, your application can quickly return responses to users, creating a more seamless experience.
- Prioritize Jobs:
Not all background jobs are created equal. Some tasks are critical and need to be processed immediately, while others can wait. Laravel’s queue system allows you to assign priorities to different queues, ensuring that the most important tasks are handled first.
By specifying which queue a job should be placed on, you can control the execution flow of your tasks. For instance, you can create high-priority queues for critical operations, such as processing payments or real-time notifications, and lower-priority queues for less urgent jobs, like sending confirmation emails or processing bulk data.
$job = (new ProcessPodcast($podcast)) ->onQueue('high') // Assigning to the 'high' priority queue ->delay(now()->addMinutes(10)); // Delaying job execution by 10 minutes
- Horizontal Scaling of Queues:
As your application grows and starts handling more traffic, the volume of background jobs will also increase. To prevent bottlenecks and ensure jobs are processed efficiently, it's important to scale your queue workers horizontally. Laravel’s queue system makes it easy to scale by running multiple workers on separate servers or processes, enabling you to process more jobs concurrently.
For example, in a high-traffic SaaS application, a single queue worker may not be able to handle the load. By adding more workers across multiple servers or containers, you can increase the throughput of your job processing. Laravel’s queue system allows you to scale your workers as needed to handle the increasing volume of jobs without sacrificing performance.
To start multiple workers, you can run several queue worker processes:
php artisan queue:work --queue=high --daemon php artisan queue:work --queue=low --daemon
This allows you to run different workers for different queues, processing high-priority tasks on one server and low-priority tasks on another. Additionally, you can use queue supervisors like Supervisor (Linux process control tool) or Horizon (Laravel's specialized queue dashboard) to monitor, scale, and restart workers as needed.
4. Optimize Middleware and Route Handling
Middleware is an essential part of Laravel’s routing system, but too much middleware on every request can slow things down.
- Limit Middleware Usage:
Only apply middleware where necessary. For example, don’t load authentication middleware for routes that don’t require user login.
Route::middleware(['auth'])->group(function () { Route::get('/dashboard', [DashboardController::class, 'index']); });
- Use Route Caching:
As mentioned before, Laravel offers route caching to reduce the overhead of bootstrapping routes every time they are requested. For a large SaaS application with many routes, this can lead to significant performance gains.
php artisan route:cache
5. Optimize File and Asset Handling
Handling large assets like images, videos, and other files can significantly impact server performance if not managed correctly.
-
Use a CDN (Content Delivery Network):
One of the most effective ways to optimize file handling is to offload static assets like CSS, JavaScript, images, fonts, and other media files to a Content Delivery Network (CDN).
CDNs distribute your static assets across multiple servers located in various geographic regions, allowing users to download files from a server that’s physically closest to them. This reduces latency, speeds up page load times, and minimizes the load on your origin server, which can focus on processing dynamic requests.
Cloudflare or Amazon Cloudfront are some of the best CDN options out there. -
Image Optimization:
Images are often some of the heaviest assets loaded on a page, and unoptimized images can severely impact page load times. Optimizing your images reduces their size without compromising quality, ensuring faster load times and better user experiences.-
Compress images: Use tools like Laravel’s Intervention/Image or external services for dynamic image resizing and compression.
use Intervention\Image\Facades\Image; $image = Image::make('path/to/image.jpg') ->resize(800, 600) ->encode('jpg', 75); // Compress the image with 75% quality
- WebP Format: Consider serving images in WebP format, which offers better compression than traditional formats like JPEG or PNG. Laravel packages like spatie/laravel-image-optimizer can help automate this process.
-
6. Optimize the Laravel Application
While Laravel is designed to be relatively lightweight and efficient out of the box, there are several key optimizations you can make to enhance its performance, particularly for high-traffic SaaS applications.
-
Use OPcache:
One of the simplest and most effective ways to boost Laravel’s performance is by enabling PHP’s OPcache. OPcache improves performance by caching the compiled bytecode of PHP scripts, meaning that scripts don't need to be parsed and compiled on every request. This can dramatically reduce request times and server load, particularly for applications with high traffic, where every millisecond matters.
To enable OPcache on a typical Ubuntu server, you can run the following command:sudo apt install php-opcache
-
Deploy with Octane:
To install Octane, you can run the following commands:
For applications that require even more speed and scalability, Laravel Octane is a game changer. Octane boosts Laravel’s performance by using modern servers like Swoole or RoadRunner. These servers keep the application in memory between requests, which eliminates the need for bootstrapping the framework on every request, leading to a significant reduction in request latency.
Unlike traditional PHP applications where every request starts from scratch (loading configurations, bootstrapping the framework, etc.), Octane ensures that much of this work is already done, resulting in lightning-fast response times. This is particularly useful for SaaS applications that handle thousands of requests per second.
composer require laravel/octane php artisan octane:install php artisan octane:start
-
Consider FrankenPHP for Even Greater Performance:
Another emerging technology that can supercharge your Laravel application is FrankenPHP, a modern application server built on top of the Caddy web server. FrankenPHP is designed to offer exceptional performance and scalability by compiling your PHP application into a Go-powered application, enabling persistent connections and extremely fast request handling.
FrankenPHP can significantly reduce the overhead traditionally associated with PHP applications by skipping the PHP engine’s repeated bootstrapping processes. It supports Laravel out of the box, making it an attractive alternative for developers looking to take performance optimization to the next level. - Optimize Composer Autoloading:
By using Composer’s optimize-autoloader flag, you can minimize the autoloading overhead in production environments, leading to faster class loading times.
composer install --optimize-autoloader --no-dev
7. Monitor and Scale Your Infrastructure
Even with a highly optimized codebase, scaling your infrastructure to meet the demands of increasing traffic is crucial for maintaining performance and availability in high-traffic SaaS applications. Monitoring your infrastructure in real-time and making use of elastic scaling features ensures your application remains responsive as user demand grows.
-
Horizontal Scaling: Distribute the Load:
As your traffic increases, a single server will eventually become a bottleneck, no matter how well-optimized your application is. Horizontal scaling solves this by distributing traffic across multiple servers, preventing any single server from becoming overwhelmed.
To distribute traffic effectively, you can set up load balancing, which ensures that incoming requests are spread evenly across a cluster of servers. This approach improves fault tolerance, as if one server fails, the load balancer can direct traffic to another healthy server. -
Database Replication: Scale Reads Horizontally:
For large-scale SaaS applications, databases can become the primary source of bottlenecks. Database replication is a powerful strategy to scale your infrastructure horizontally by splitting read and write operations across different servers. In a replicated database setup:
- The primary database handles write operations.
- Read replicas are used to handle read operations, distributing the load and allowing more read queries to be processed concurrently.
This is particularly useful for high-traffic SaaS applications where a large volume of users may be fetching data simultaneously. By replicating the database, you prevent your primary server from becoming overwhelmed with read queries, allowing it to focus on write operations. -
Auto-Scaling: Adapt to Traffic Spikes:
While manual scaling can help you prepare for high traffic, there will be times when demand spikes unexpectedly. Auto-scaling allows your infrastructure to dynamically adjust to traffic in real-time, adding or removing servers as needed to maintain optimal performance.
Cloud platforms like AWS, Google Cloud, and DigitalOcean provide built-in auto-scaling capabilities. These services automatically spin up new instances when CPU usage, memory, or other metrics reach a certain threshold, ensuring that your application can handle increased traffic without intervention.
Conclusion
The myth that Laravel can't handle high-traffic SaaS applications is just that—a myth. By using the right techniques, from efficient database queries to advanced caching, queuing systems, and infrastructure scaling, Laravel can scale just as well as any other modern framework. The key to success lies in understanding the nuances of performance optimization and continually fine-tuning your application as it grows.
Ultimately, it’s not the framework that limits your scalability, but how you use it. Laravel provides a solid foundation with powerful tools to build scalable, performant applications—allowing you to focus on what really matters: delivering value to your users.
So don’t let the naysayers hold you back—optimize wisely, and watch your Laravel SaaS soar, even under the heaviest of traffic.
Keep building. 🤘