PHP 8.4: Exciting New Features!
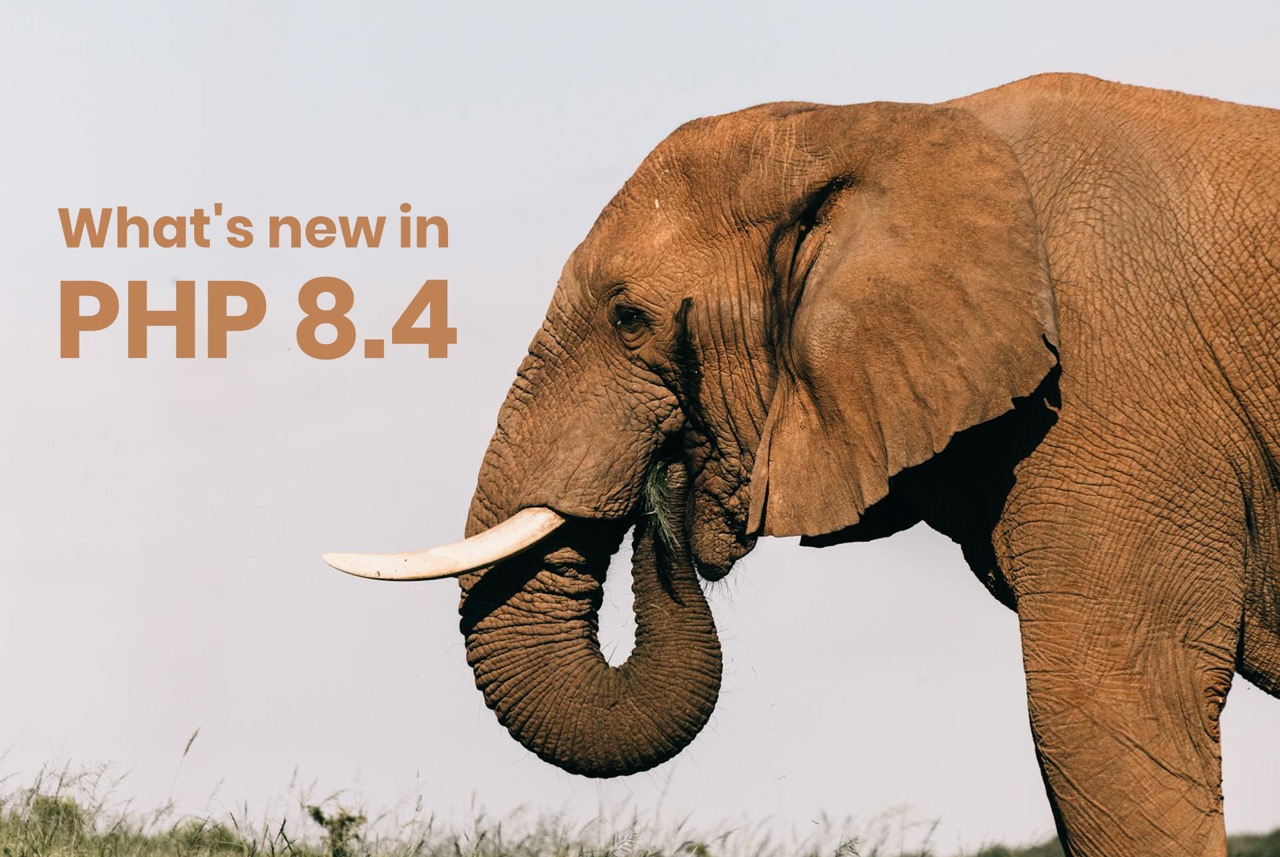
As we gear up for the release of PHP 8.4 on November 21, 2024, it’s hard not to look back and appreciate how far the language has come. For those who’ve been around since the days of PHP 5 or even earlier, each new version feels like a reunion with an old friend who’s grown in ways you never expected.
And PHP 8.4? It’s a big one. Lots of exciting new features that I personally can't wait to use in my projects!
Let’s take a look into those!
Property Hooks: Goodbye Boilerplate, Hello Elegance
Writing getters and setters for every property can quickly become cumbersone. Enter property hooks, perhaps the most significant evolution in PHP since the introduction of typed properties.
Now, properties can manage their own get and set hooks directly, cutting down repetitive code and making classes cleaner and more intuitive:
class AuthorViewModel
{
public string $displayName {
set (string $name) {
$this->displayName = str_replace(' ', '', $name);
}
get => $this->displayName
}
}
What's more, hooks can now be defined directly in interfaces, a feature that might take some time to get used to!
interface Eatable
{
public int $calories { get; }
public string $type { get; set; }
}
"New" Without Parentheses: Small Change, Big Impact
A subtle yet impactful change in PHP 8.4 allows chaining methods on new objects without requiring parentheses.
Old way:
$url = (new PaymentProvider($name))->getRedirectUrl();
New way:
$url = new PaymentProvider($name)->getRedirectUrl();
Asymmetric Visibility: Controlling Access Like Never Before
PHP has traditionally allowed developers to control the visibility of object properties by defining them as public, private, or protected. However, this visibility has always been symmetric, meaning the same access level applies to both reading (get) and writing (set) operations.
This change aims to introduce asymmetric visibility, enabling developers to specify different visibility levels for reading and writing a property. Inspired by Swift’s approach, this enhancement offers greater flexibility and fine-grained control over property access in PHP.
For example, making a property publicly readable but writable only within the class:
class Library
{
public private(set) string $catalogName;
}
The first access modifier is "public", which means the field is public, but "private(set)" mean that you can edit it only from within the "private" context, which means "only from within that class".
Want to allow write access to subclasses instead? Easy:
class UserProfile
{
public protected(set) string $emailAddress;
}
And this works with promoted properties as well:
public function __construct(
public private(set) Gamer $gamer;
) {}
Array Find: Finally, a Native Solution
For years, PHP developers relied wither on themselves or on external libraries for basic collection operations. But PHP 8.4 brings us the long-awaited array_find() and its companions, like array_any() and array_all().
$firstLargeImage = array_find($images, fn($img) => $img->size > 500);
HTML5 Support: Parsing the Modern Web
Remember when parsing HTML in PHP meant wrestling with quirks of DOMDocument? Now, with the new \Dom\HTMLDocument class, PHP 8.4 introduces proper HTML5 support, making it easier to work with and parse web pages natively.
$htmlDoc = \Dom\HTMLDocument::createFromString('<div>Hello, world!</div>');
This new parser ensures that handling complex HTML5 structures is no longer a chore.
Exit and Die as Proper Functions
The exit and die commands have been treated more like magical keywords than proper functions.
With PHP 8.4, exit() and die() are now properly recognized as functions. It's worth noting that the keyword variant without parentheses still behaves the same way.
The #[Deprecated] Attribute
Once upon a time, we relied on docblocks to mark code as deprecated. Now, PHP gives us the #[Deprecated] attribute, which allows metadata like this:
#[Deprecated("Use updatedMethod() instead", since: "2.0")]
function legacyMethod() { }
Pretty handy, isn't it?
Lazy Objects: Native Support for Proxies
PHP 8.4 introduces native support for lazy objects, improving a pattern frequently used in frameworks to create proxy objects. With this feature, developers can defer the initialization of objects until they are actually needed, improving performance in resource-intensive applications.
Here’s how you can use lazy objects in PHP 8.4:
$initializer = static fn(MyClass $proxy): MyClass => new MyClass(123);
$reflector = new ReflectionClass(MyClass::class);
$object = $reflector->newLazyProxy($initializer);
This approach allows you to define a callback that initializes the object only when accessed. Native support for lazy objects eliminates the need for custom proxy implementations, reducing boilerplate code while enhancing maintainability.
This feature is particularly valuable in scenarios involving dependency injection containers, ORM entities, and other areas where object instantiation may be expensive or unnecessary until certain conditions are met.
Deprecated: Implicit Nullable Types
PHP 8.4 addresses a longstanding inconsistency with implicit nullable types. Previously, a typed variable with a default null value would automatically be treated as nullable, which could lead to unexpected behavior. For example:
function save(Post $post = null) {}
This implicit conversion of the $post parameter to a nullable type is now deprecated and will be removed in PHP 9. The recommended solution is to explicitly define nullable types which avoids ambiguity:
function save(?Post $post = null) {}
Conslusion
PHP 8.4 introduces a range of features aimed at simplifying development, reducing boilerplate and improving readability.
Obviously, PHP is not dying, but rather it continues to grow stronger every year! :)
I'm personally looking forward to integrating these features into my projects, and I encourage you to explore them and make the most of what PHP 8.4 has to offer.
Keep building! 🤘