Automate Code Styling: Using Laravel Pint with Git Pre-Commit Hooks
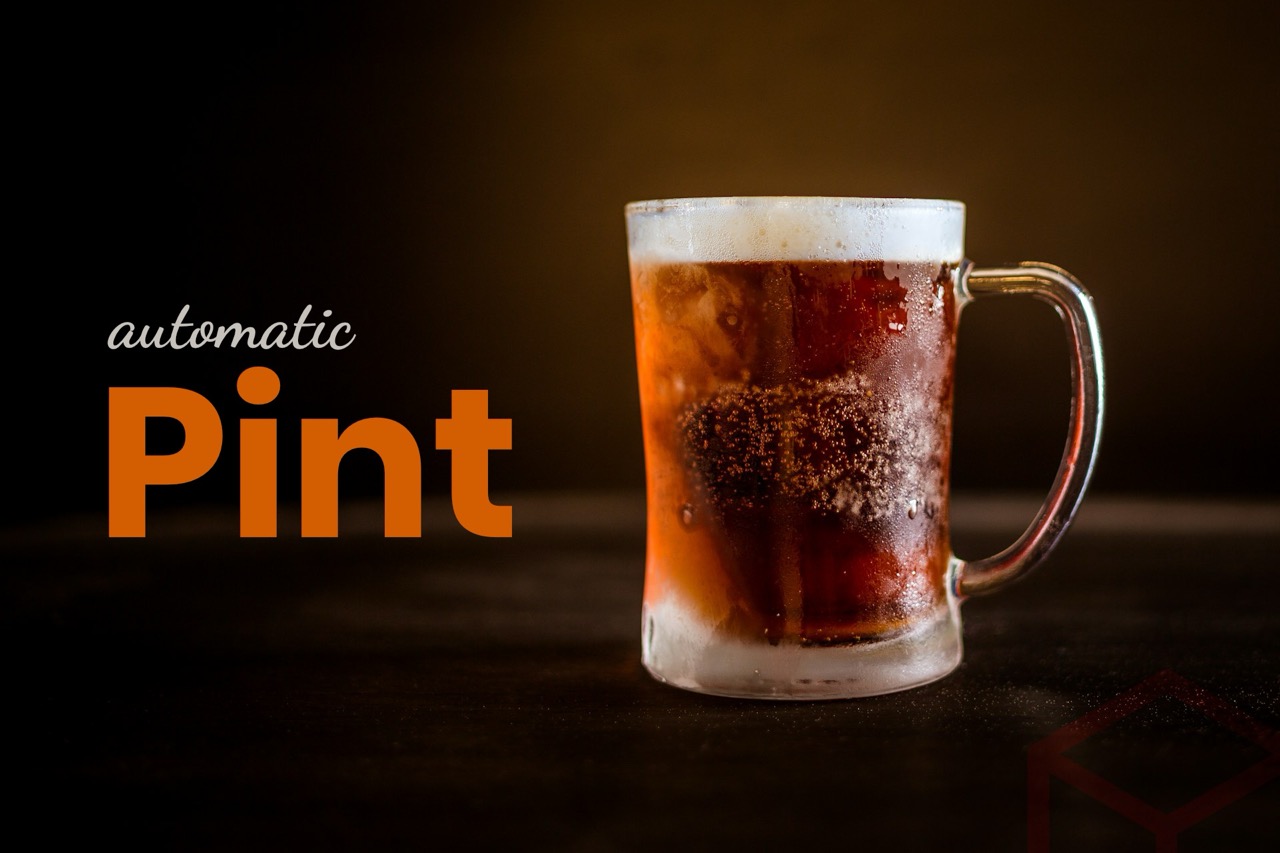
Code formatting is an essential part of maintaining a clean and readable codebase. Laravel Pint, a zero-configuration code styling tool for PHP, helps ensure your code adheres to consistent style rules. To automate this process, you can integrate Laravel Pint with a Git pre-commit hook, preventing non-compliant code from being committed.
Step 1: Install Laravel Pint
First, install Laravel Pint as a development dependency in your project:
composer require laravel/pint --dev
Once installed, you can verify its functionality by running:
vendor/bin/pint
This will analyze and fix your PHP files according to Laravel’s default coding style.
Step 2: Create the Git Pre-Commit Hook
Git hooks allow you to run scripts before or after certain Git actions. To enforce Laravel Pint formatting before committing, create a pre-commit hook.
Navigate to your project's Git hooks directory, then run:
mkdir -p .git/hooks
Create a new file for the pre-commit hook:
touch .git/hooks/pre-commit
chmod +x .git/hooks/pre-commit
Open the .git/hooks/pre-commit
file in a text editor and add the following script:
#!/bin/sh
# Run Laravel Pint to fix code style issues
echo "Fixing code style with Laravel Pint..."
staged_files=$(git diff --cached --name-only --diff-filter=ACM -- '*.php');
vendor/bin/pint $staged_files -q
git add $staged_files
This script will pick all the .php files that are being committed, format them using Pint, then do the actual commit.
Step 3: Test the Pre-Commit Hook
To verify the hook is working correctly:
-
Make an intentional code style violation in a PHP file.
-
Stage the file using
git add .
. -
Try committing the changes:
git commit -m "Test Pint pre-commit hook"
If Pint detects any formatting issues, it will fix them before committing.
Conclusion
By integrating Laravel Pint with a Git pre-commit hook, you ensure that every commit adheres to Laravel's coding standards, reducing code review friction and maintaining a clean codebase. This simple automation can help teams enforce consistency and improve overall code quality.
Keep Building! 🚀