๐ค๏ธ User Onboarding
SaaSykit comes with a Filament plugin (Filament Onboarding) that helps you onboard your users by collecting some information from them before getting started with your application.
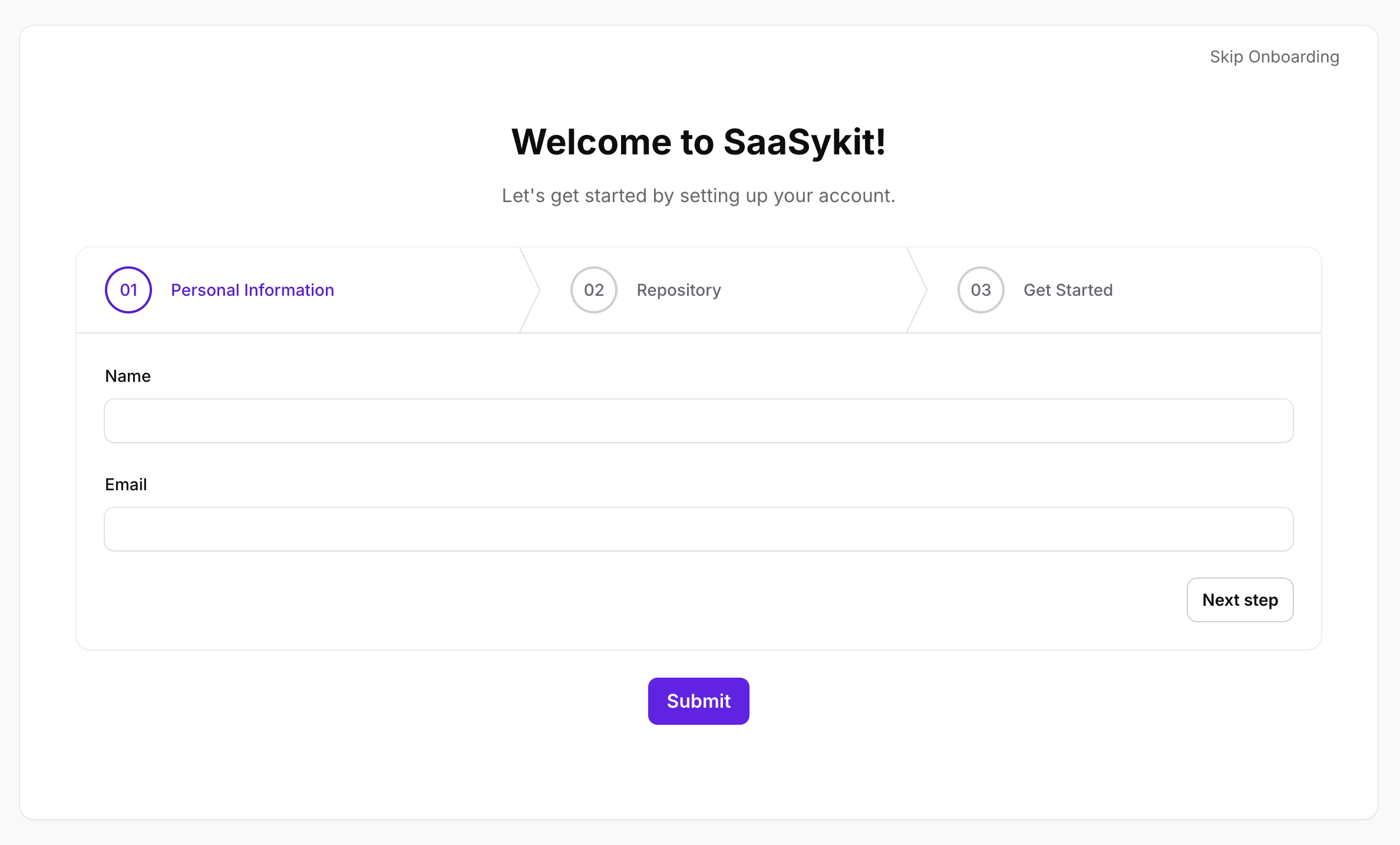
Features of Filament Onboarding in a nutshell:โ
- Hands off onboarding: Just pass in the Livewire form component and the plugin will take care of the rest.
- Supports single & multi-tenant applications: Works with both versions of SaaSykit (SaaSykit & SaaSykit Tenancy)
- Keeps track of who completed the onboarding: This is useful for tracking who completed the onboarding and who didn't.
- Mandatory onboarding: You can force users to complete the onboarding before they can access the application.
- Allows users to skip the onboarding: If you don't want to force users to complete the onboarding, you can allow them to skip it.
Installationโ
To use the plugin, you'd need to add it to your project via composer:
- First you need to include the repository in your
composer.json
file as this is a private package:
"repositories": [
{
"type": "vcs",
"url": "https://code.saasykit.com/saasykit/filament-onboarding.git"
}
]
- Then, you can install the package via composer:
composer require saasykit/filament-onboarding
- You'd need to publish and run the migrations with:
php artisan vendor:publish --tag="filament-onboarding-migrations"
php artisan migrate
- (Optional) In case you need to customize them, you can publish the views using:
php artisan vendor:publish --tag="filament-onboarding-views"
Usageโ
Once you've installed the package, you can start using it by adding the FilamentOnboardingPlugin
to your Filament
plugin list in your DashboardPanelProvider
(located in app/Providers/Filament/DashboardPanelProvider.php
):
use Saasykit\FilamentOnboarding\FilamentOnboardingPlugin;
class DashboardPanelProvider extends PanelProvider
{
public function panel(Panel $panel): Panel
{
return $panel
// some other configurations
->plugins([
FilamentOnboardingPlugin::make(), // Add this line
]);
}
}
Adding the plugin to your Filament
plugin list will automatically show an empty form when you visit {site-url}/dashboard/onboarding
.
You can customize the form by creating a Livewire form and passing it to the plugin. Actually it's best to overwrite the Saasykit\FilamentOnboarding\Pages\OnboardingPage
class for that.
Create a new Filament page (let's call it CustomOnboardingPage
). Make sure to add the page to your User Dashboard (not the admin panel), and add the following code to it:
<?php
namespace App\Filament\Dashboard\Pages;
use Filament\Forms\Components\Actions\Action;
use Filament\Forms\Components\TextInput;
use Filament\Forms\Components\Wizard;
use Saasykit\FilamentOnboarding\Pages\OnboardingPage;
class CustomOnboardingPage extends OnboardingPage
{
public string $name;
public string $email;
protected function getFormSchema(): array
{
return [
Wizard::make([
Wizard\Step::make('Personal Information')
->schema([
TextInput::make('name'),
TextInput::make('email'),
]),
Wizard\Step::make('Repository')
->schema([
// ...
]),
Wizard\Step::make('Get Started')
->schema([
// ...
]),
])->nextAction(
fn (Action $action) => $action->label('Next step')->color('gray')->outlined(),
)
];
}
public function submit()
{
// $this->validate();
// SAVE THE SETTINGS HERE
$this->onboarded(); // don't forget to call this method to mark the user/tenant as onboarded
}
}
Make sure to edit the getFormSchema
method to add all the fields you need in your onboarding form. You can use a Wizard component to create a multi-step form like above, or just use a simple form with all the fields in one step.
You can add the logic to save the settings in the submit
method.
Don't forget to call the onboarded
method to mark the user/tenant as onboarded.
Then, you can add the CustomOnboardingPage
to your DashboardPanelProvider
:
use Saasykit\FilamentOnboarding\FilamentOnboardingPlugin;
use App\Filament\Dashboard\Pages\CustomOnboardingPage;
class DashboardPanelProvider extends PanelProvider
{
public function panel(Panel $panel): Panel
{
return $panel
// some other configurations
->plugins([
FilamentOnboardingPlugin::make()
->onboardingPage(CustomOnboardingPage::class),
]);
}
}
That's it! Now when you visit {site-url}/dashboard/onboarding
, you'll see your custom onboarding form.
Multi-tenant panelsโ
If you're using the multi-tenant version of SaaSykit, you can add the FilamentOnboardingPlugin
to your TenantPanelProvider
as well, set the ->multiTenancy(true)
method to the plugin:
use Saasykit\FilamentOnboarding\FilamentOnboardingPlugin;
class TenantPanelProvider extends PanelProvider
{
public function panel(Panel $panel): Panel
{
return $panel
// some other configurations
->plugins([
FilamentOnboardingPlugin::make()
->multiTenancy(true),
]);
}
}
The plugin will track the onboarding of the Tenant as a whole (not the user).
Mandatory onboardingโ
If you want to force users to complete the onboarding before they can access the application, you can set the ->mandatoryOnboarding(true)
method to the plugin:
use Saasykit\FilamentOnboarding\FilamentOnboardingPlugin;
class DashboardPanelProvider extends PanelProvider
{
public function panel(Panel $panel): Panel
{
return $panel
// some other configurations
->plugins([
FilamentOnboardingPlugin::make()
->mandatoryOnboarding(true),
]);
}
}
By setting onboarding as mandatory, users will be redirected to the onboarding page when they try to access any page on the dashboard.
When the user (or tenant) has already completed the onboarding, they will not be redirected to the onboarding page and will be able to access the dashboard normally.
Skipping onboardingโ
If you don't want to force users to complete the onboarding, you can allow them to skip it by setting the ->skippable(true)
method to the plugin:
use Saasykit\FilamentOnboarding\FilamentOnboardingPlugin;
class DashboardPanelProvider extends PanelProvider
{
public function panel(Panel $panel): Panel
{
return $panel
// some other configurations
->plugins([
FilamentOnboardingPlugin::make()
->skippable(true),
]);
}
}
By setting onboarding as skippable, users will see a "Skip" button on the onboarding page that will allow them to skip the onboarding and access the dashboard (and that will also be stored in the database).
Redirection after onboardingโ
By default, after the user completes the onboarding, they will be redirected to the dashboard. If you want to redirect them to a different page, you can set the ->afterOnboardingRedirectTo()
method to the plugin:
use Saasykit\FilamentOnboarding\FilamentOnboardingPlugin;
class DashboardPanelProvider extends PanelProvider
{
public function panel(Panel $panel): Panel
{
return $panel
// some other configurations
->plugins([
FilamentOnboardingPlugin::make()
->afterOnboardingRedirectTo('/custom-page'),
]);
}
}
Enabling onboarding using a callableโ
If you want to enable onboarding based on a condition, you can pass a callable to the ->enabledUsing()
method of the plugin.
For example, for tenant onboarding, you can enable onboarding only if the current user is a tenant admin (has a specific permission):
use Saasykit\FilamentOnboarding\FilamentOnboardingPlugin;
use App\Services\TenantPermissionService;
class TenantPanelProvider extends PanelProvider
{
public function panel(Panel $panel): Panel
{
return $panel
// some other configurations
->plugins([
FilamentOnboardingPlugin::make()
->enabledUsing(function (TenantPermissionService $service) {
return $service->tenantUserHasPermissionTo(Filament::getTenant(), auth()->user(), TenancyPermissionConstants::PERMISSION_UPDATE_TENANT_SETTINGS);
})
]);
}
}
Tracking onboarding completionโ
You can use the Saasykit\FilamentOnboarding\Services\OnboardingManager::isOnboarded()
or Saasykit\FilamentOnboarding\Services\OnboardingManager::isOnboardedOrSkipped()
method to check if the user (or tenant) has completed the onboarding or not.
Going live (Production)โ
As the plugin is private, you might find it useful to fork the plugin and host it on your own Github repository. You can then include the repository in your composer.json
file like this:
"repositories": [
{
"type": "vcs",
"url": "[email protected]:{YOUR_GITHUB_USERNAME}/filament-onboarding.git"
}
]